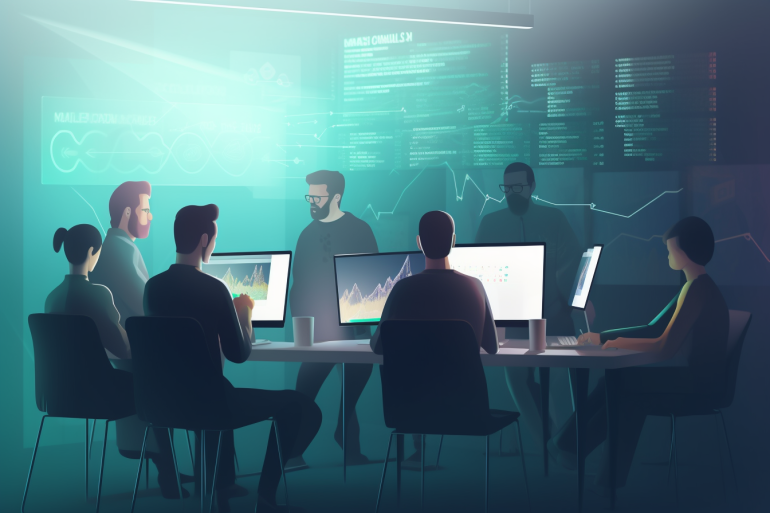
React.js Interview: Top 100 Questions by Levels
Whether you’re a React.js developer or looking to hire the best talent, this one will interest you. We’ve collected 100+ questions & tasks to run during a React.js tech interview crafted for different experience levels. We hope it helps you prepare for a smooth process as an interviewer or an interviewee.
Junior React.js engineer
Javascript fundamentals
1 Can you explain what JavaScript is?
2 What are the data types supported by JavaScript?
3 Can you explain how variables are declared in JavaScript?
4 What are JavaScript operators?
5 What is the difference between “==” and “===” operators?
React basics
6 Why would you choose to use React?
7 Can you explain what a React Component is?
8 What is JSX?
React advanced
9 What is “prop drilling,” and how do you handle it?
React libraries and tools
10 How do you use “create-react-app”?
ES6 and beyond
11 What is ES6, and how is it different from ES5?
12 What is destructuring, and how do you use it in JavaScript?
Functional programming
13 Can you explain what functional programming is?
14 What is a pure function?
React router
15 Can you explain how to set up basic routing in a React application?
Styled components
16 Can you explain how to use basic styles with styled components?
TypeScript
17 What is TypeScript, and how does it differ from JavaScript?
18 Can you explain how to declare a variable in TypeScript?
GraphQL
19 Can you explain how to make a basic query in GraphQL?
Testing (Jest, Enzyme)
20 What is unit testing?
21 What is Jest, and how do you use it?
Performance optimization
22 What is performance optimization, and why is it important?
23 Can you list some ways to improve performance in a React app?
Design patterns
24 What is a design pattern?
25 Can you explain the concept of ‘props’ in React?
Web accessibility
26 What is web accessibility, and why is it important?
27 Can you list some ways to make a website more accessible?
Practical tasks
28 Create a simple React component with a greeting message like “Hello, World!”.
29 Create a component with a state that changes over time (for example, a counter).
30 Set up a simple Redux store and write a reducer.
31 Convert a function to an arrow function.
32 Implement a basic routing system with at least two routes in React.
33 Write a styled component.
34 Write a basic GraphQL query.
Middle React.js engineer
JavaScript fundamentals
1 How does JavaScript handle asynchronous operations?
2 What is a callback function in JavaScript, and how does it work?
3 How does ‘this’ keyword work in JavaScript?
React basics
4 How does React’s Virtual DOM work?
5 What are React hooks, and how do you use them?
6 Can you explain how “props” work in React?
React advanced
7 What is the difference between “useEffect” and “useLayoutEffect”?
React libraries and tools
8 How does Redux Middleware work?
ES6 and beyond
9 What is the spread operator, and how would you use it?
10 Can you explain the concept of generators in JavaScript?
Functional programming
11 How would you use higher-order functions in JavaScript?
React router
12 What is the difference between ‘BrowserRouter’ and ‘HashRouter’?
13 How would you pass data through React Router?
Styled components
14 Can you explain how to use themes with Styled Components?
TypeScript
15 What are Interfaces in TypeScript, and how do you use them?
GraphQL
16 What is a Mutation in GraphQL, and how does it work?
17 Can you explain how to use variables in GraphQL queries?
Testing
18 What is Enzyme, and how do you use it to test React components?
Performance optimization
19 How does ‘shouldComponentUpdate’ help in optimizing React apps?
20 What tools would you use to find performance issues in your code?
Design patterns
21 Can you explain the concept of ‘higher order components’ in React?
Web accessibility
22 What is ARIA, and how would you use it?
23 How can you ensure color accessibility on a webpage?
Practical tasks
24 Implement a closure in JavaScript.
25 Write a piece of code that demonstrates hoisting in JavaScript.
26 Write a Class Component and a Functional Component. Both should render the same output.
27 Implement a simple context and use it in a component.
28 Write a Redux Thunk function for asynchronous actions.
29 Write a block of code showing the difference between ‘var,’ ‘let,’ and ‘const.’
30 Write an immutable function.
31 Create a styled component that changes its style based on props.
32 Define a type for a function that accepts two numbers and returns a number.
33 Write a mock for a simple JavaScript function.
34 Convert a component into a higher-order component.
Senior React.js engineer
JavaScript fundamentals
1 What is Prototype-based inheritance in JavaScript?
2 How does garbage collection work in JavaScript?
3 Explain how to use Promises in JavaScript.
4 Can you explain how error handling works in JavaScript?
React basics
5 How would you optimize an extensive React.js application for performance?
6 Can you explain the lifecycle methods in a React component?
7 How would you test React components?
React advanced
8 How would you implement Code Splitting in a React application?
9 Can you explain how Concurrent Mode works in React?
React libraries and tools
10 Can you explain how Redux Saga is used?
ES6 and beyond
11 Can you explain what a Proxy is in JavaScript?
12 How does a JavaScript Set differ from an Array?
Functional programming
13 Can you explain the concept of currying in JavaScript?
React router
14 Can you explain how to navigate using React Router programmatically?
Styled components
15 What are the performance implications of using Styled Components?
16 How can you use global styles with Styled Components?
TypeScript
17 Can you explain how to use generics in TypeScript?
GraphQL
18 How would you handle caching with GraphQL?
Testing
19 How would you test async functions?
Performance optimization
20 Can you explain the concept of ‘lazy loading’ and how it can help improve performance?
Design patterns
21 Can you explain the concept of ‘render props’ in React?
Web accessibility
22 How would you make a single-page application accessible?
23 Can you explain how to set up an accessibility audit?
Practical tasks
24 Write an asynchronous function and show how the event loop handles this function.
25 Implement a component that uses error boundaries to catch errors in child components.
26 Discuss an example where you’ve implemented Server-Side Rendering (SSR) in React.
27 Compare two situations: one where you used Redux and one where you used Context API. Which one was more suitable and why?
28 Refactor a piece of callback-based asynchronous code using async/await.
29 Write two functions that can be composed together.
30 Implement a 404-page using React Router.
31 Imagine you have a JavaScript project. What steps would you take to migrate it to TypeScript?
32 What steps would you take if you had to set up a GraphQL server?
33 Write a test case for a simple React component.
34 Write a React component that demonstrates the use of memorization.
35 Implement a compound component.